In this episode we dive deep into complex investigation about different kind of scams in clearnet. As title suggest, it involves not so legal services advertised on old, almost unused message board.
TL;DR
We will write code to scrape links to fake shops, run WHOIS against it, extract info (email, phone numbers, names), make a Maltego graph and look for connections to use it in next part of the research.
Maltego mentioned my blog in category "7 OSINT Websites & Blogs You Must Know", so as a thanks, we will use Maltego to show initial connections between the websites.
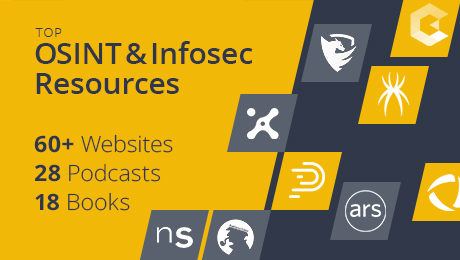
Introduction
Old message boards/forums, especially ones without proper security measures, are perfect target for spammers. You often see spam comments on obsolete websites mostly due to poor security like lack of Captcha. This time, we will focus on one discussion board - voy.com
voy.com is another free message board and was created around 2000. As with this type of services often happens, it's not updated since at least 2016. Even in 2001, forum was full of spam in each board, you can check it in Wayback Machine. Below is an example from one board that contains scams about earning easy money.

Yes, you can find some legit discussion there, but it's a really small percentage what's really going on. It's also a place for sharing different kind of fetishes, I didn't even know some of them exist. If you want to check it out, you can just use "site:voy.com" google dork.
Voy is a service managed by Voyager Info-Systems from Beverly Hills, CA, address from the website points to Mailbox Rental Services, but their website looks amazing though.
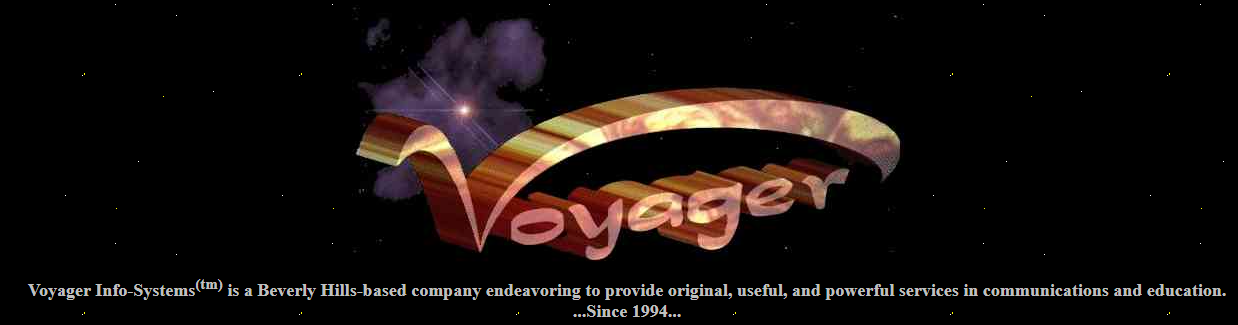
CEO of Voyager Info-Systems is Rudi Sahebi (probably Iranian), and some clues lead also to company Sunny Oasis - http://sunnyoasis.com/.
We won't dig into the company but rather the content that is being posted everyday to their message board.
Gathering data
As mentioned, Voy is full of posts offering drugs, firearms, exotic pets and prescription free pharmacies. Couple clicks on the website and looking on boards might give you below view. It looks normal on first sight
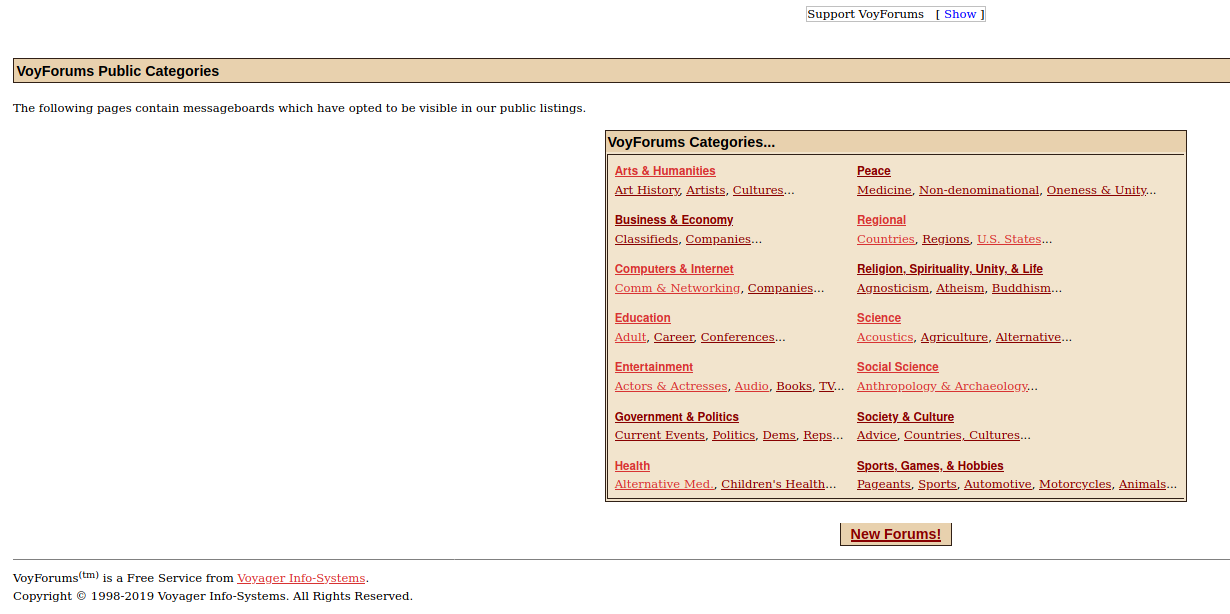
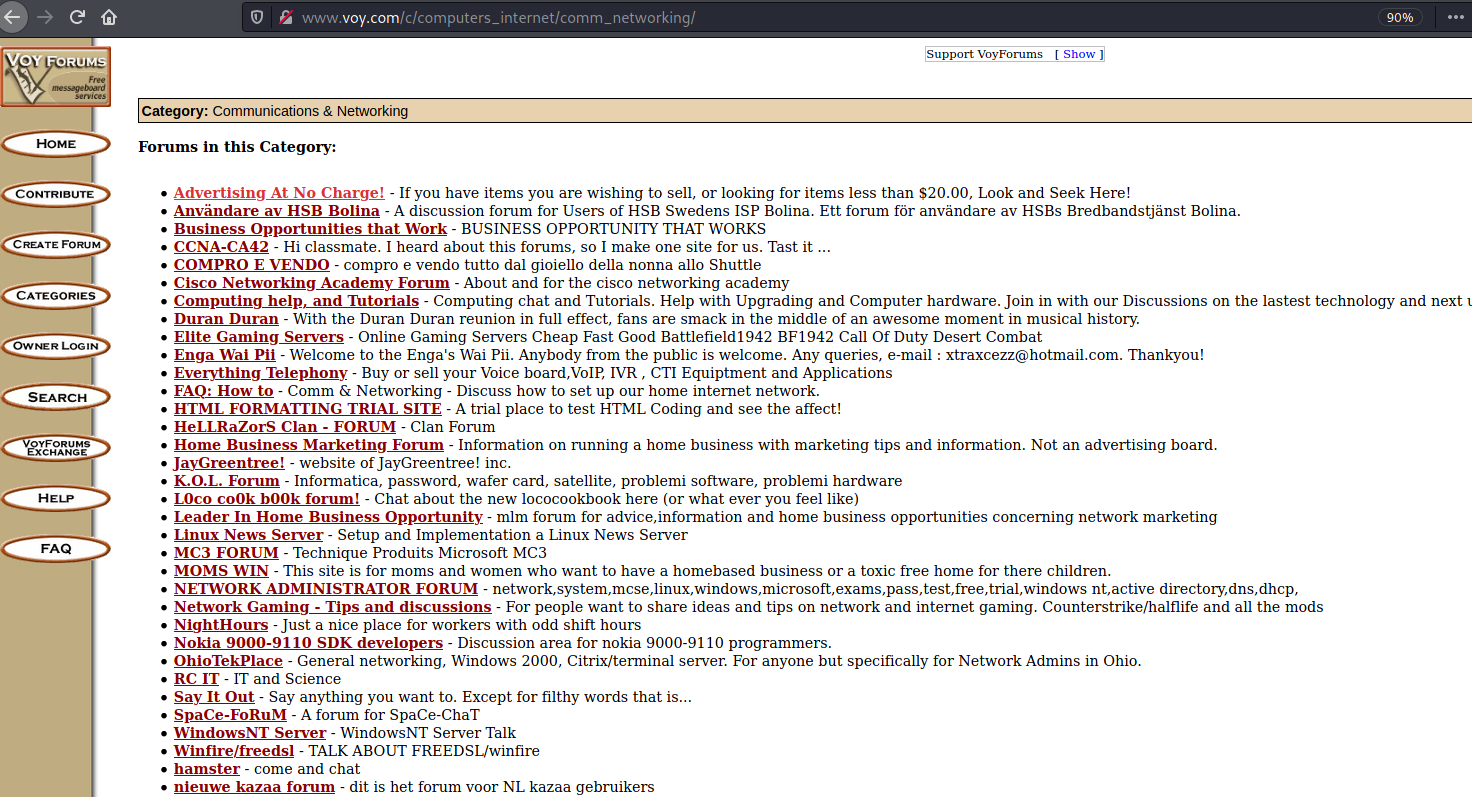
but each forum contain spam topics
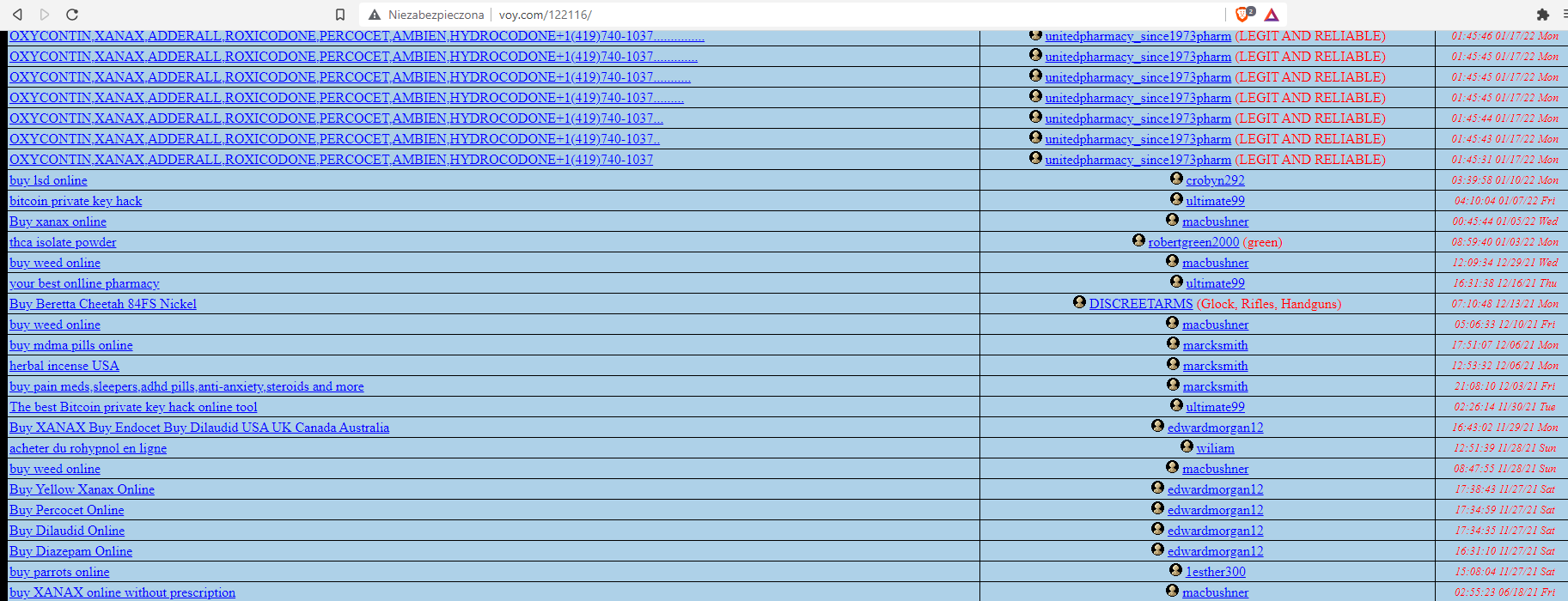
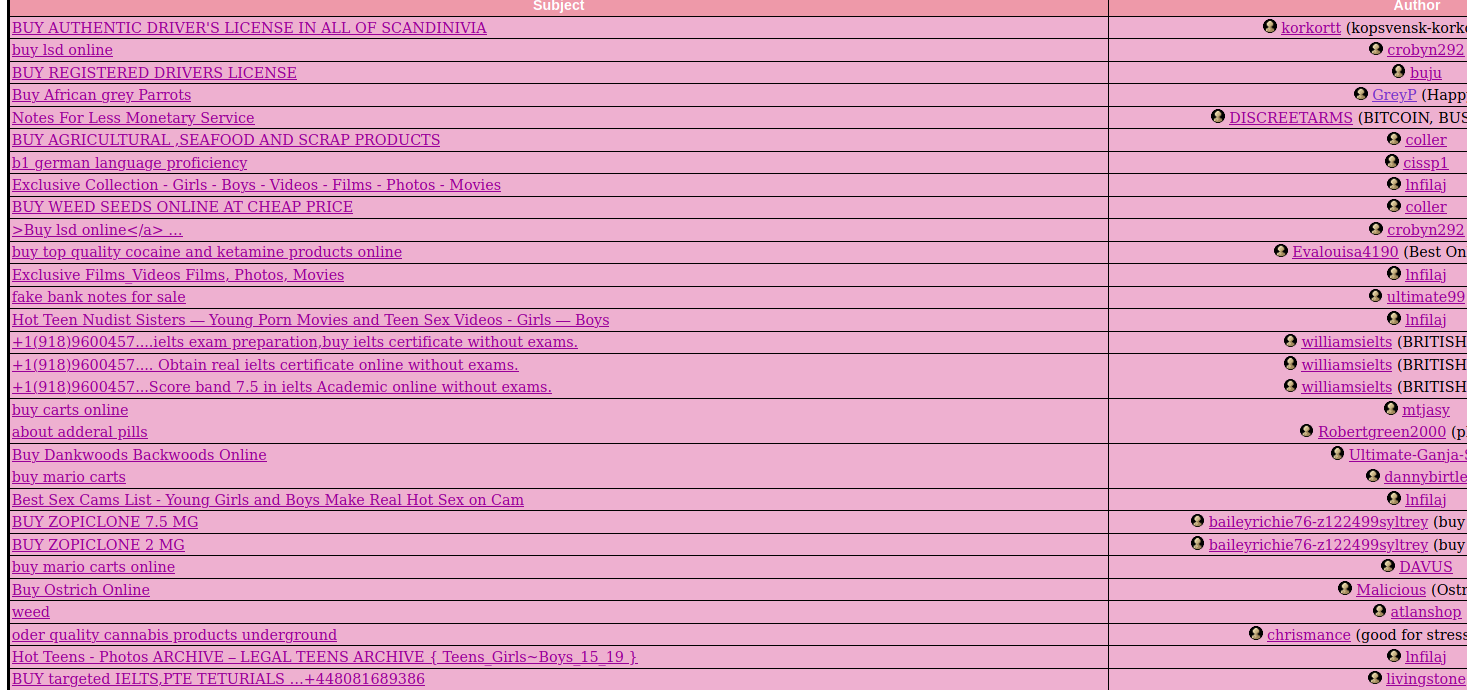
When we open some post, it contains shit loads of links to the shops. In below case it's for firearms, driving licences, exams and exotic parrots.
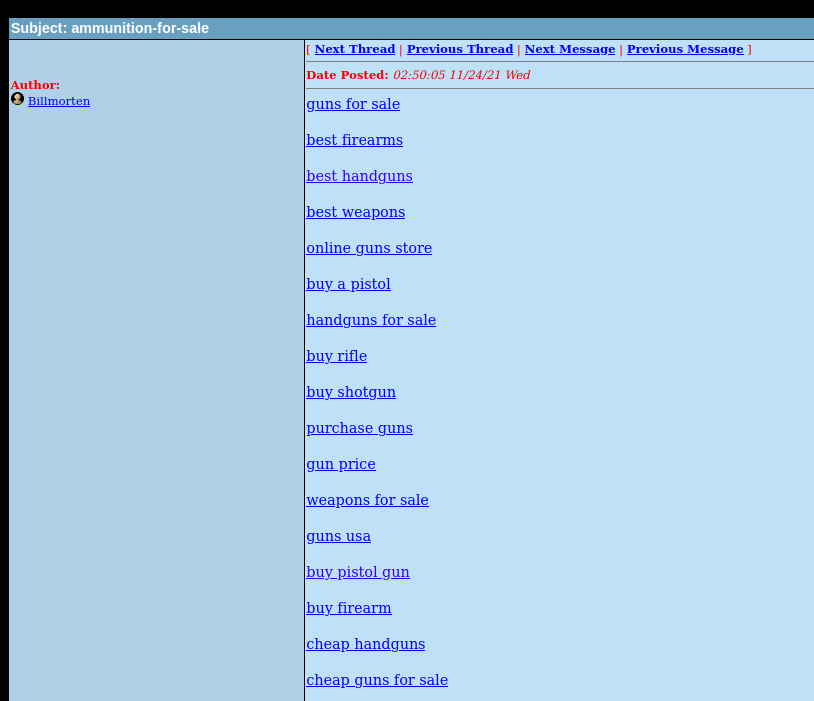
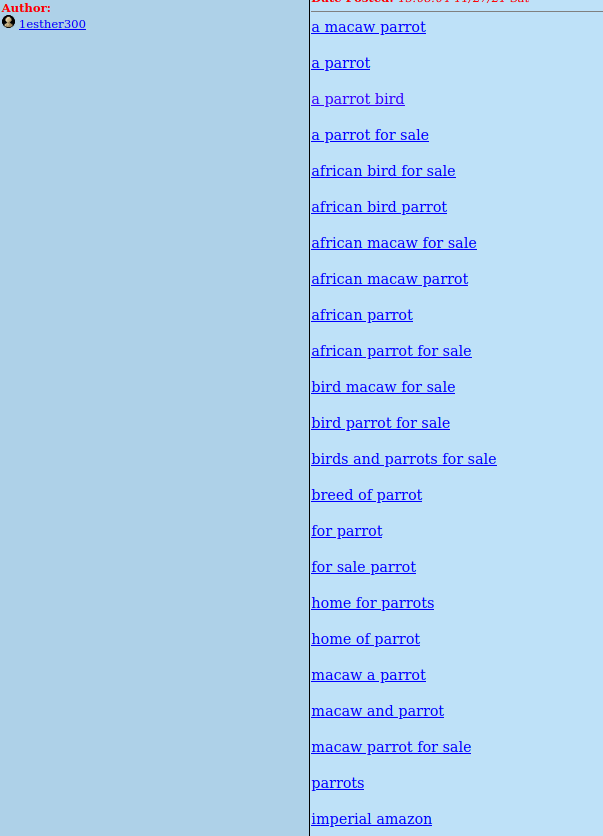
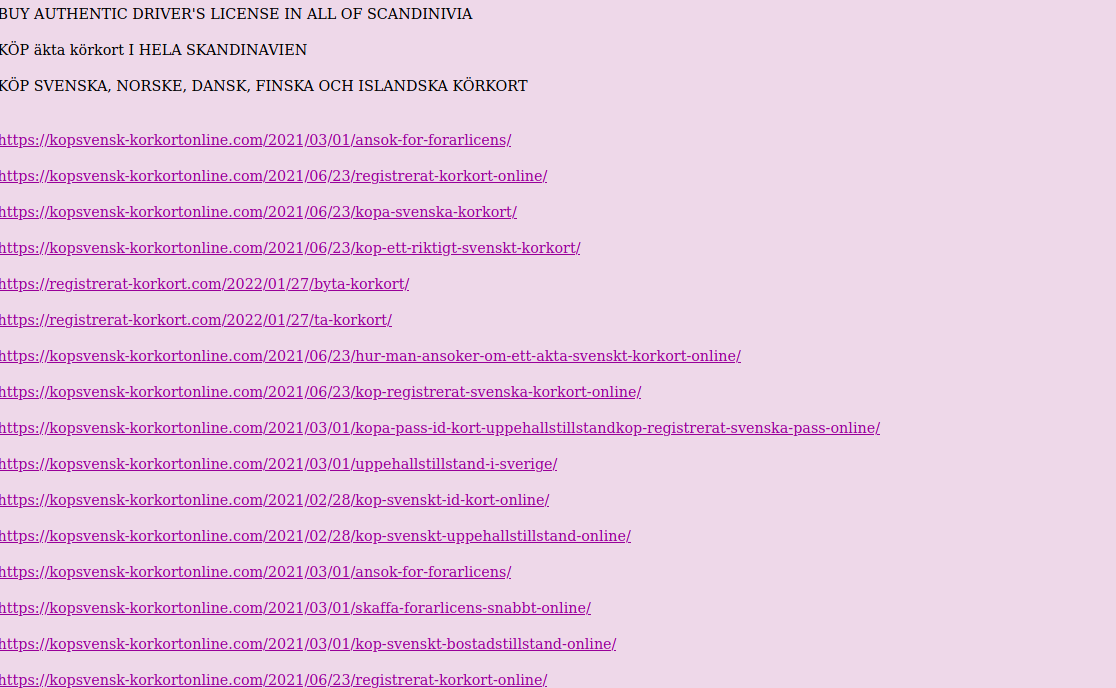
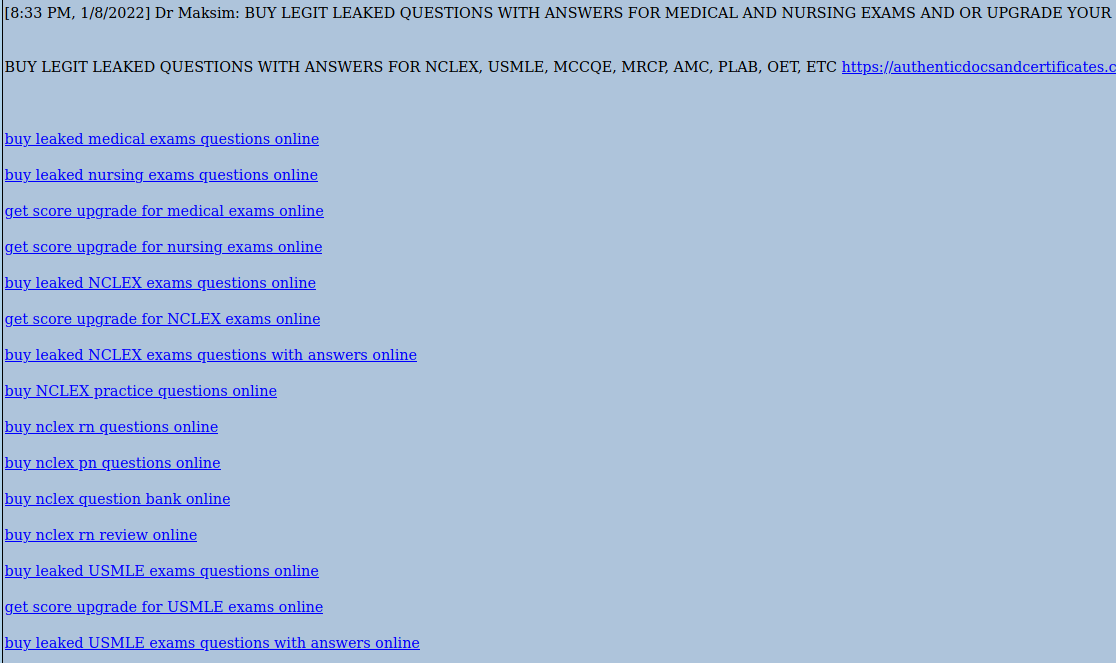
This is how it looks in majority of the boards I reviewed, however on all of them these same links were posted. It means, someone might use automation script to publish the content.
At the end, urls bring us to the variety of shops. They all are very similar, same wordpress template, themes and photos. You can buy heroin, passports, counterfeit bills, firearms, OSCP certificate or exotic reptiles.
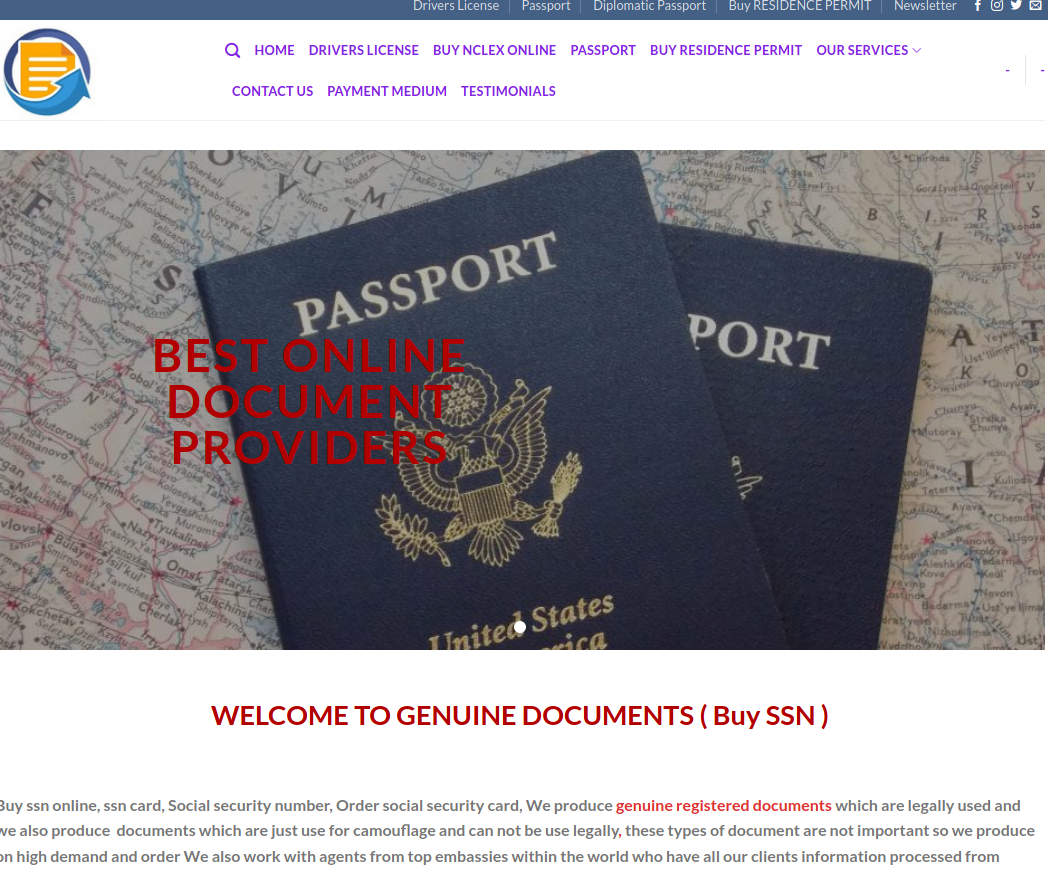
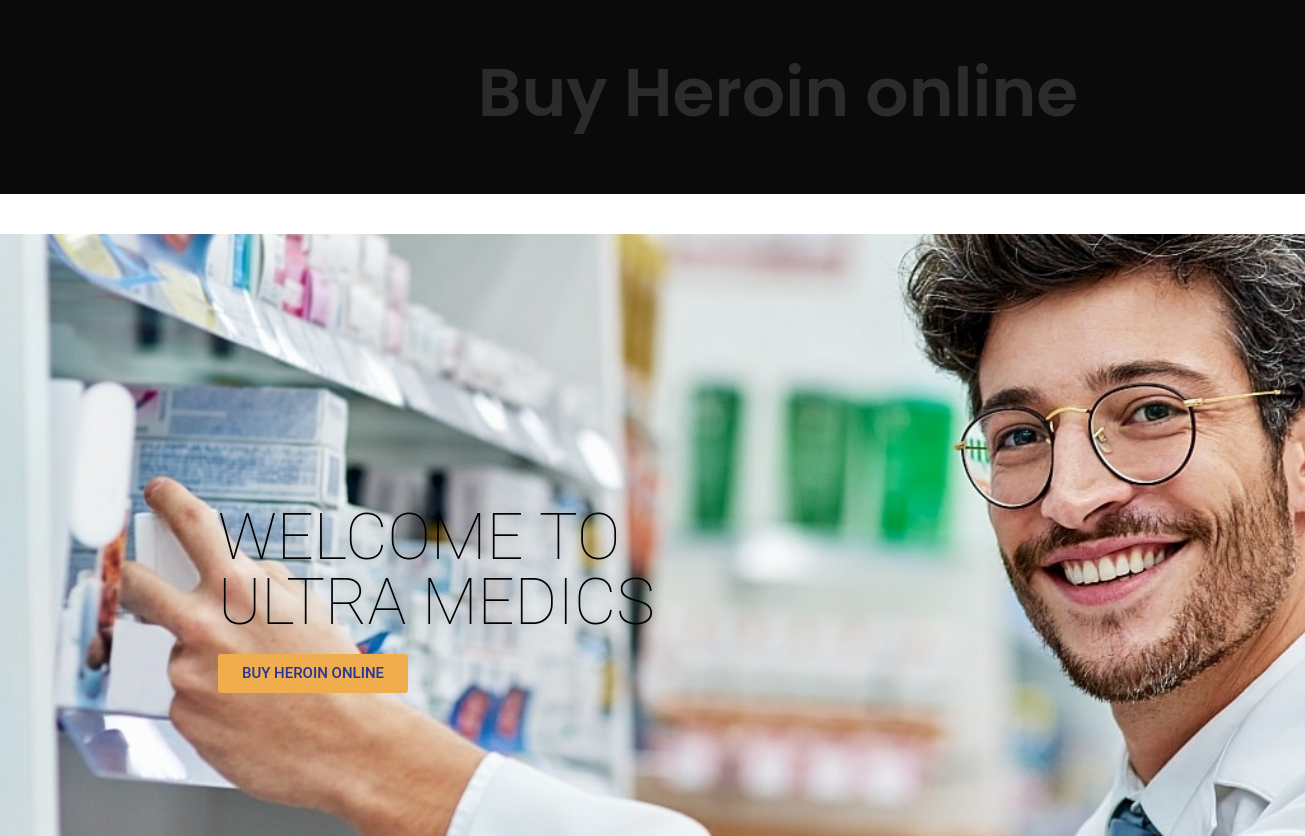
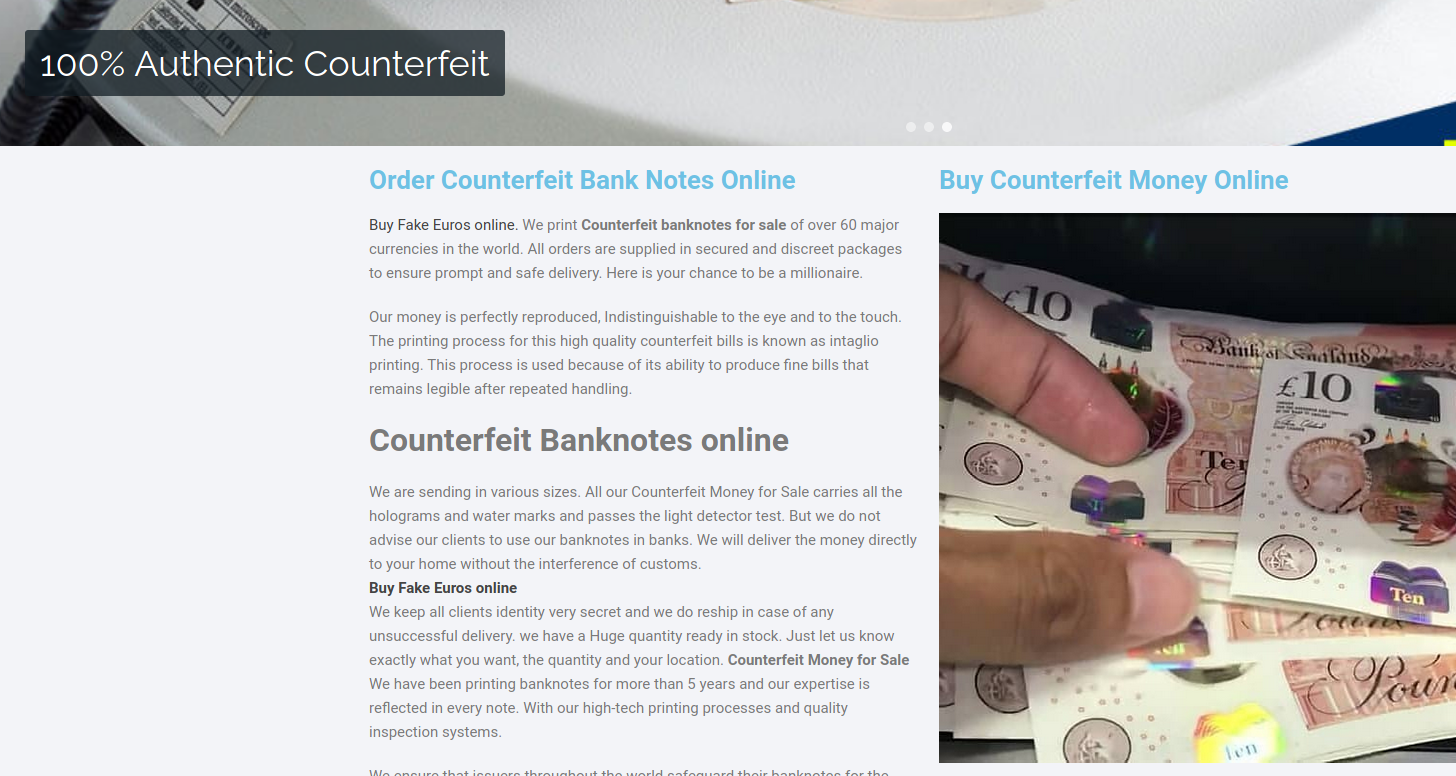
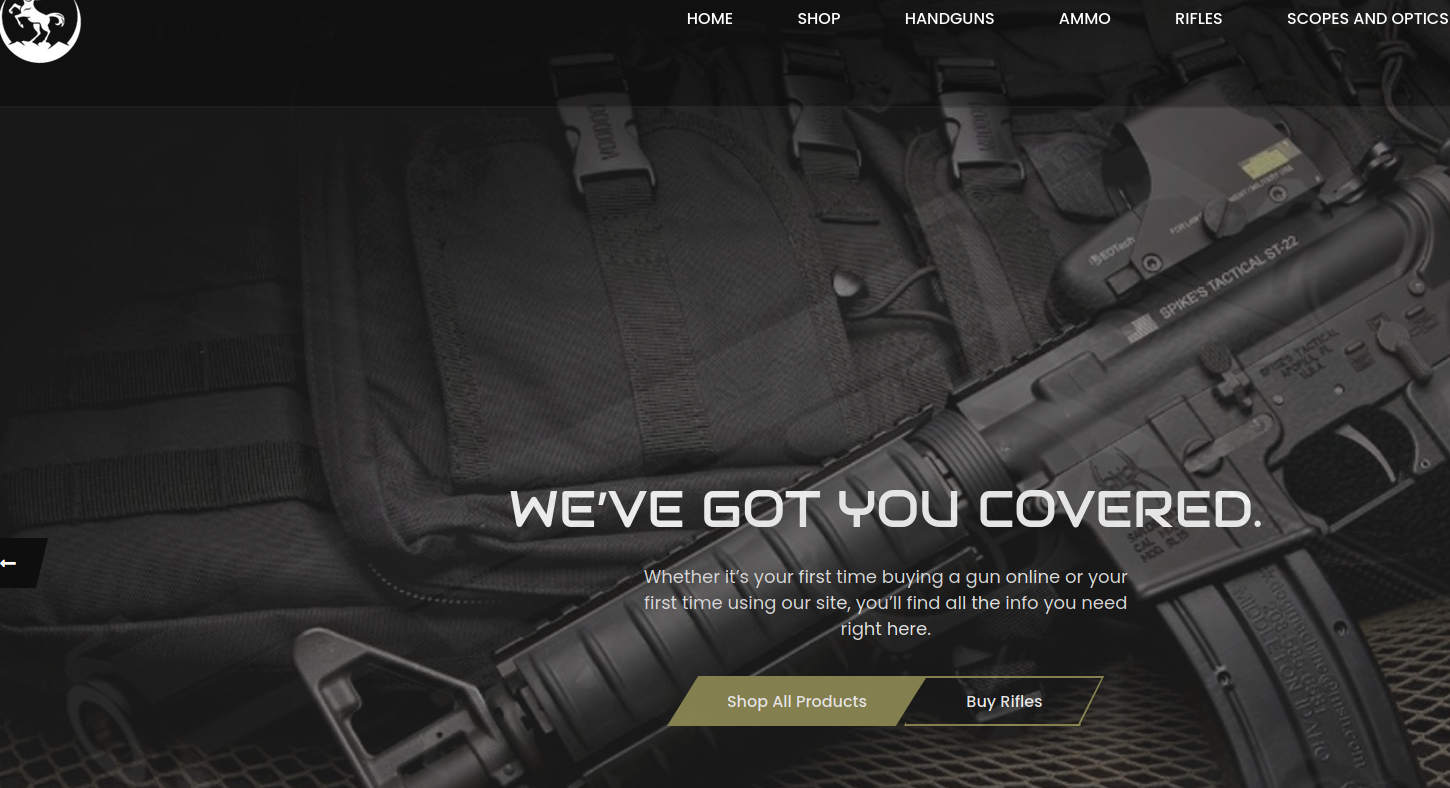
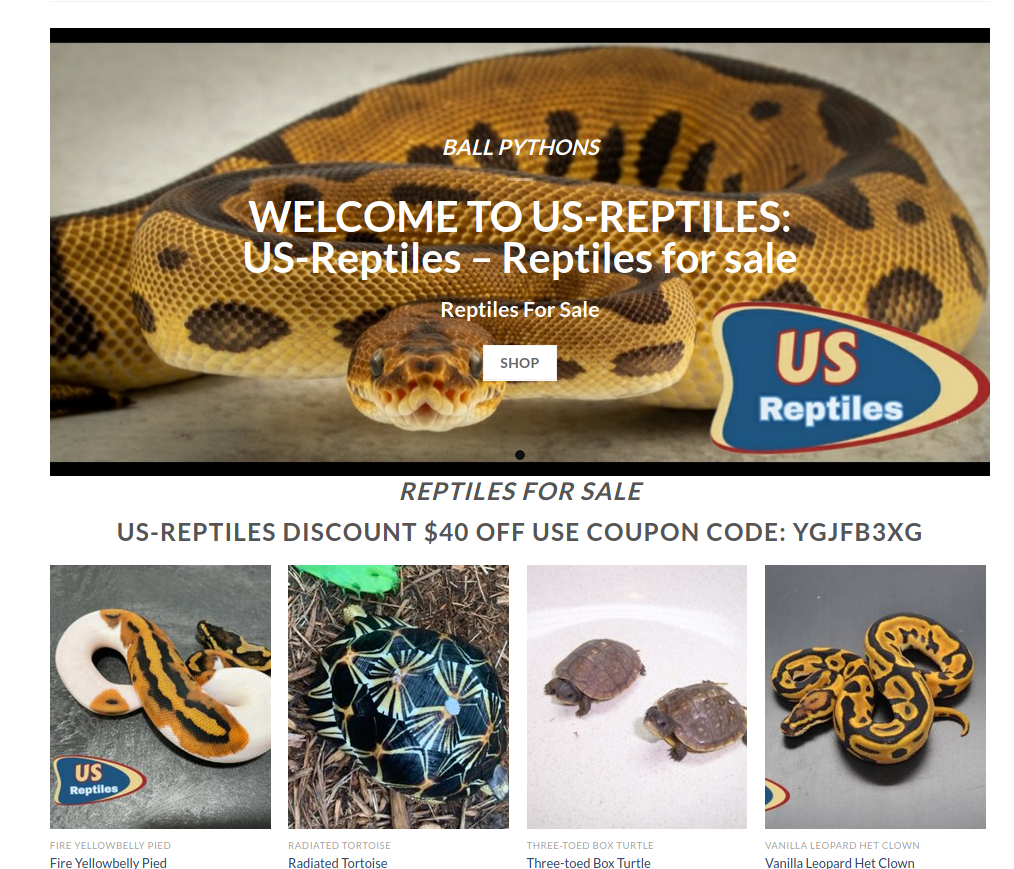
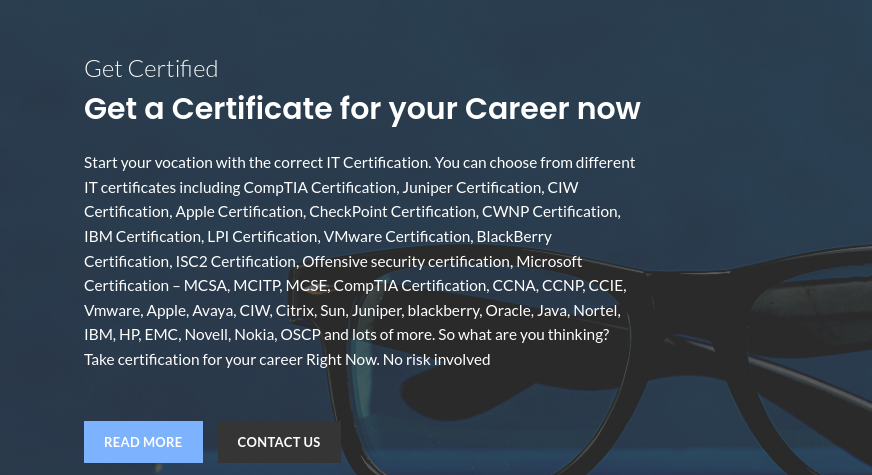
It's obvious non-delivery scams offering illegal goods, you can spot plenty of them in clearnet as well as in darknet. It's a good tactic for scammers because victim won't go to police and admit he tried to order heroin from some shady store, but unfortunately many people still fall for it.
Do all of the websites have something in common? We will try to answer this question at the end of the article, but first things first - we have to collect as many links, to fraudulent sites, as possible.
To achieve this, we need to build a crawler for each board and later grab only external links posted by scammers.
We will use Python and we need:
Modules
import requests
from bs4 import BeautifulSoup
import re
from urllib import parse
Url of the board
url = "http://www.voy.com/182192/"
After getting familiar with structure of the website, you can find that each topic in board contains href parameter that ends with [digits].html. To extract only these values, we write something like below
url = "http://www.voy.com/182192/"
req = requests.get(url)
soup = BeautifulSoup(req.content)
# looking for topic link
for link in soup.find_all('a', href=True):
href = re.match(r'^([\s\d]+).html', link.attrs['href'])
if href:
domains = scrape_content(url + href.string)
for domain in domains:
print(domain)
Urls of the topics are passed here to the function 'scrape_content" which is responsible to extract only links to the fraudulent shops. I discovered that every link posted have "rel" attribute, so it was easy to distinguish them and fetch quickly.
def scrape_content(link):
unique_domains = set()
req = requests.get(link)
soup = BeautifulSoup(req.content)
for url in soup.find_all(rel=True): # find links twith "rel" attribute
domain = parse.urlsplit(url.string)
if domain.scheme:
unique_domains.add(domain.netloc)
return unique_domains
We also create a set to keep only unique domains and return only these ones. At the end we should save it to the txt file by adding to the for loop below code.
with open('domains.txt', 'a+') as f:
for link in soup.find_all('a', href=True):
...
for domain in domains:
f.write(domain + "\n")
This way we have collected all posted links in one board, which includes many topics. To scrape from other boards, just change url from the beginning.
I did this for many of the boards on the website and obtained 170 domain names providing potentially fake services. I uploaded it to Pastebin and you can find it below
pass: cVZ76YNnpU
Making connections
If you google these domains you can see that some of them were also shared in other message boards or in comments of old blogs and outdated Wordpress sites, however most of them appeared only in Voy. Posts and topics are very similar to each other - same format and way they are advertised.
So, what we can check to establish connections between our domains?
First thing that comes to mind is infrastructure, we can look where they are hosted and what registrar was used. Then, it's worth to dig deeper into IPs to see what else is using this same IP address. This way we can probably find more and more domains.
Second idea, which I will focus on, is using WHOIS records for each domain and draw connection based on email address and phone number, when available. Having such information, we can check who is behind particular shop and establish clusters of the ownership.
But, first we actually have to run our domain list against some WHOIS API. I choose, WHOISXML API which gives you 200 free credits after registration.
import requests
import json
return_dict = []
with open('domains.txt') as f: # read out domain list
for domain in f.readlines():
print(domain.rstrip())
try:
url = "https://www.whoisxmlapi.com/whoisserver/WhoisService?apiKey=[APIKEY]&outputFormat=json&&domainName=" + domain.rstrip()
r = requests.get(url)
r_json = json.loads(r.text)
return_dict.append({"name":domain.rstrip(), "registrar_name": r_json['WhoisRecord']['registrarName'],
"created":r_json['WhoisRecord']['createdDate'], "registrant":r_json["WhoisRecord"]['registrant']})
except Exception as e:
print(e)
print(json.dumps(return_dict, indent=4))
It loads our lists and access WHOISXML API to collect information about domain owner. At the end it gives json as below
{
"name": "legitchemshop.com",
"registrar_name": "NetEarth One, Inc.",
"created": "2020-01-06T18:20:36Z",
"registrant": {
"name": "james mullah",
"street1": "Southview, 73 stopford road,, flat3 st saviour,",
"city": "california",
"state": "California",
"postalCode": "94203",
"country": "UNITED STATES",
"countryCode": "US",
"email": "legitchemstore12@gmail.com",
"telephone": "12158398130",
"rawText": "Registrant Name: james mullah\n
Registrant Street: Southview, 73 stopford road,, flat3 st saviour,\n
Registrant City: california\n
Registrant State/Province: California\n
Registrant Postal Code: 94203\n
Registrant Country: US\n
Registrant Phone: +1.2158398130\n
Registrant Email: legitchemstore12@gmail.com"
}
},
{
"name": "buyoxycodoneusa.com",
"registrar_name": "NAMECHEAP INC",
"created": "2021-01-17T17:11:33.00Z",
"registrant": {
"name": "REACTIVATION PERIOD",
"organization": "Withheld for Privacy Purposes",
"street1": "Kalkofnsvegur 2",
"city": "Reykjavik",
"state": "Capital Region",
"postalCode": "101",
"country": "ICELAND",
"countryCode": "IS",
"email": "reactivation-pending@mail.withheldforprivacy.com",
"telephone": "3544212434",
"rawText": "Registrant Name: REACTIVATION PERIOD\nRegistrant Organization: Withheld for Privacy Purposes"
}
}
We have to accept that lot of domains use WHOIS protection, which means we cannot get any information out of them.
Moreover, I checked each domain separately and looked for contact details posted on the website - phone number and email address, some of them use gmail or protonmail.
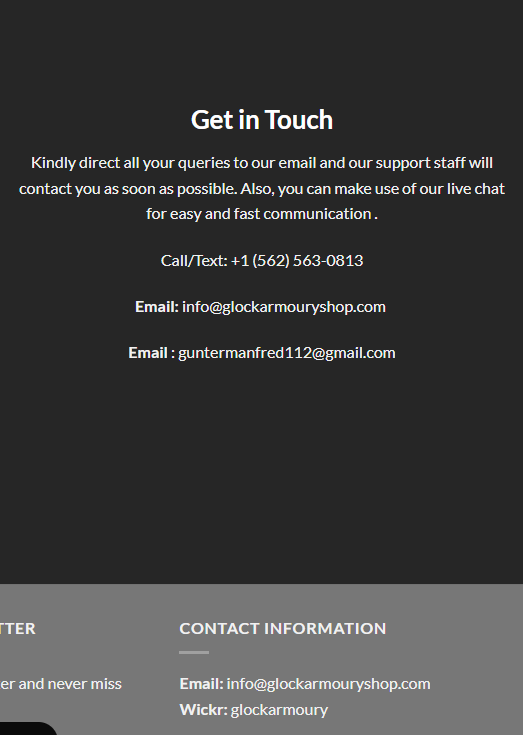
Googling these phone numbers will show that they are used in variety of scams. Different service, website, email but same phone number. Below is example, where you can buy a sex doll and contact Illuminati with only one call.
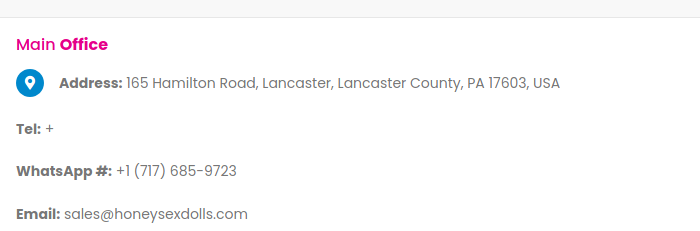

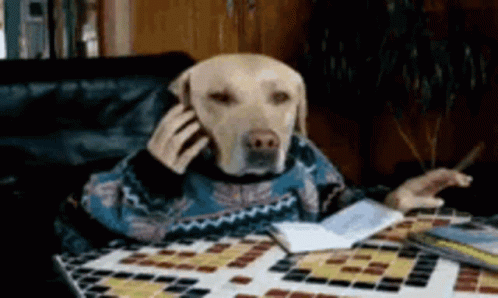
Also, glockgundealers.com and exoticpets.com, self explanatory domains, share same phone number.
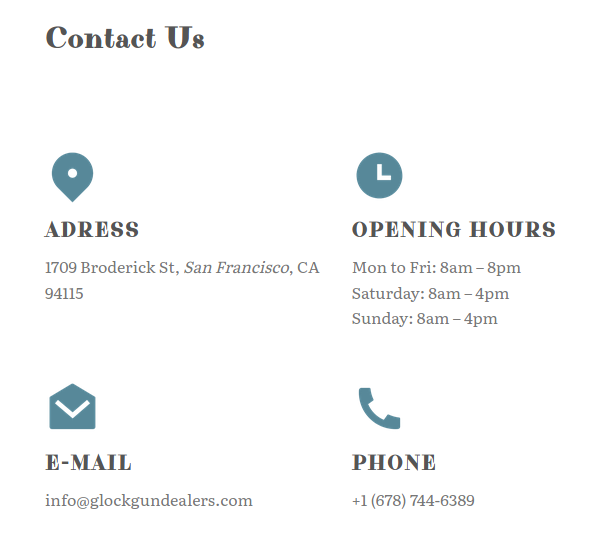
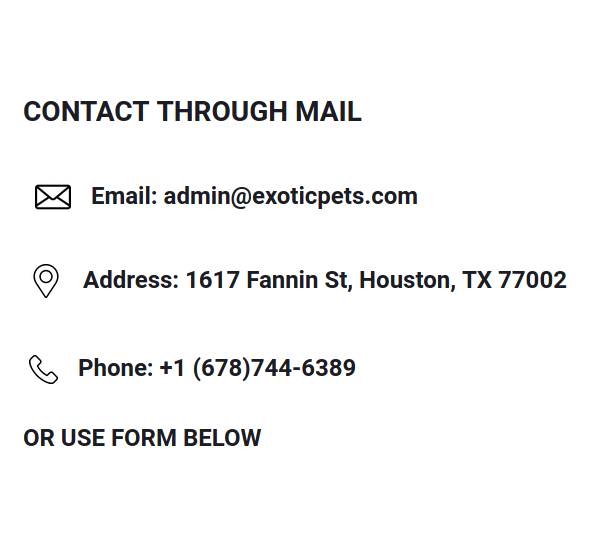
All of such manually established connections are also taken into account during investigation and visualization.
Visualization
As mentioned at the beginning, we will use Maltego to put it all together. It will allow us see the whole picture, what domains are owned by whom and if there are additional connections between them via phone number or email address.
So, I manually put the domains, that expose email address and phone number in WHOIS (from previously created json) to Maltego and run WHOISXML API transform again to show the connection. For the rest of the domains where email was found manfully on contact page, edge colour was changed to green.
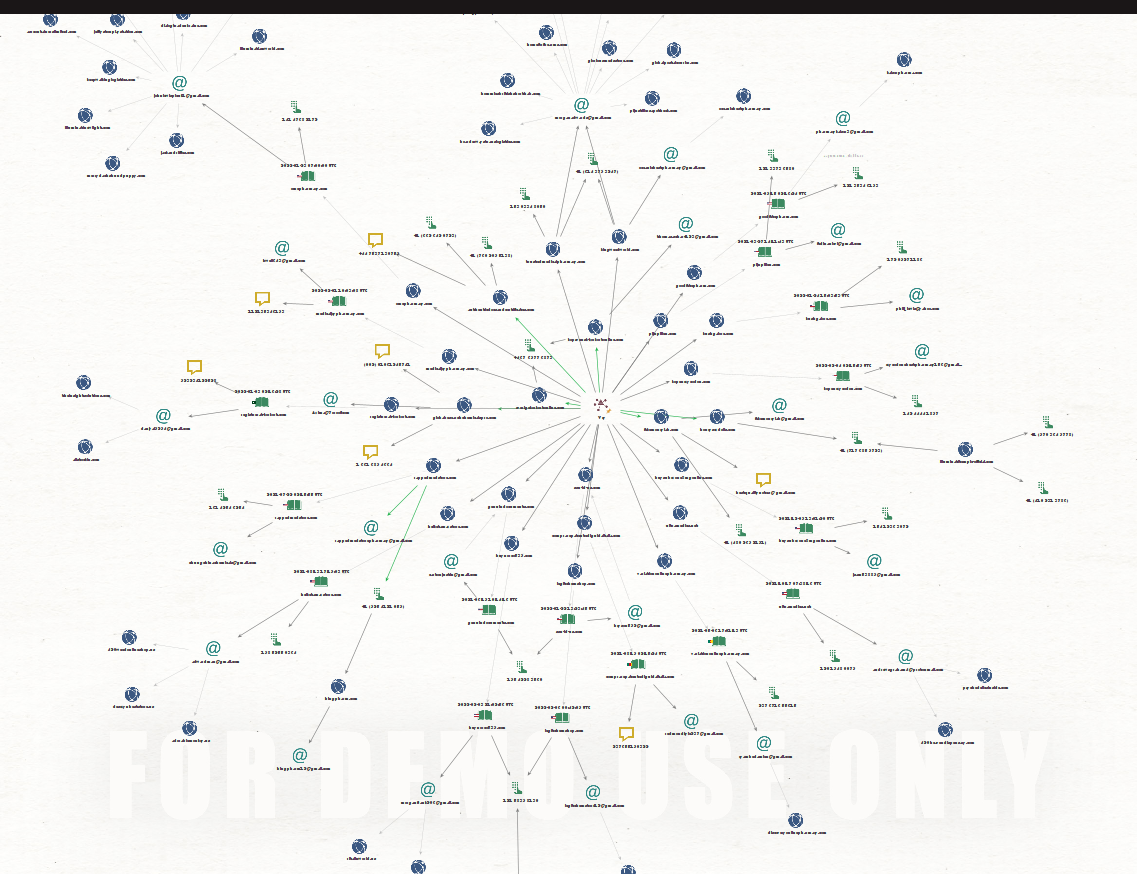
Let's take a look on some of the clusters that appears based on WHOIS information.
Here is the basic association, domain omcpharmacy.com, advertised on the forum, was registered to johnlewisplcuk1[at]gmail.com. This email address is also used in more suspicious domains and poorly tries to impersonate John Lewis & Partners.
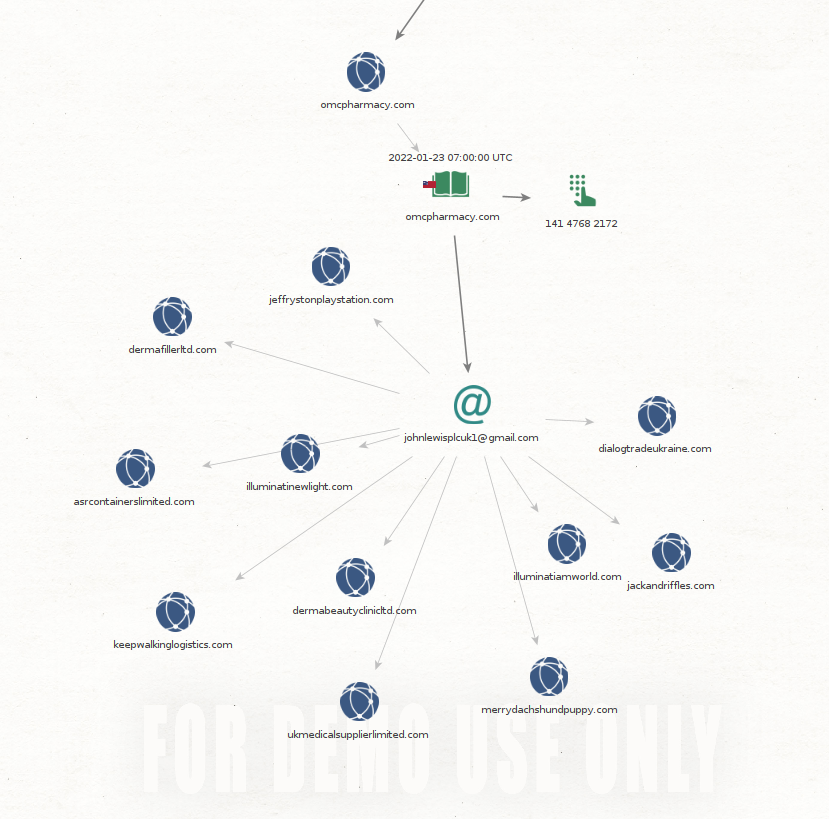
Next case is where domains have different email address but same phone number.
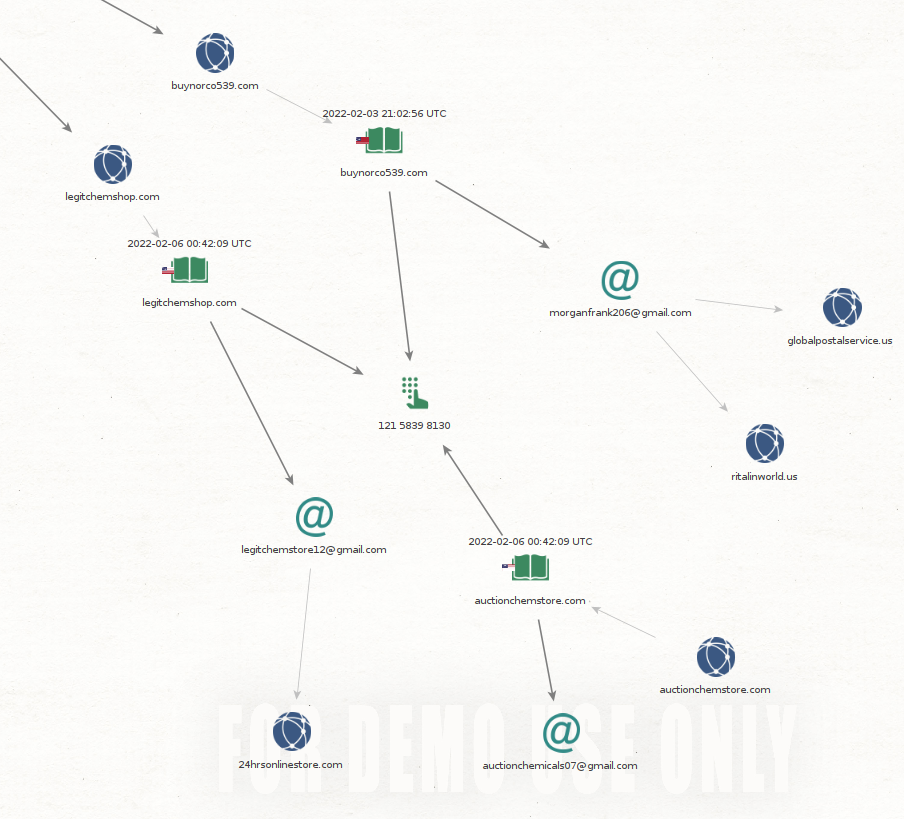
Below, two domains that allegedly provide fake documents, have same phone number but emails are completely different.
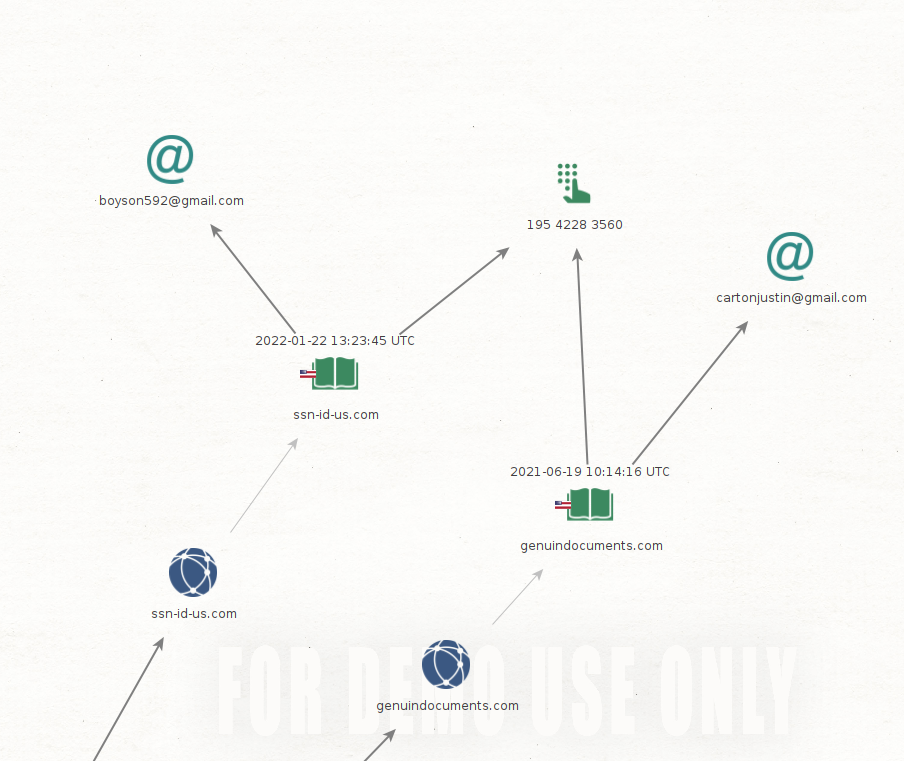
It does not end here, what brought my attention was that scammers reuse phone numbers in WHOIS and contact pages. I often see they give same email address but not the phone number, there is something phishy going definitely.
So far, we went from spam posts on some old message boards to connection graph based on WHOIS and information on the websites. The most intriguing thing are repetitive phone numbers, and in next part we will focus specifically on this matter. Will try to deanonymize people behind the numbers and look for more connections to feed our investigation.
Summary
Non-delivery scams still work, no matter what products are offered, even illegal ones, from exotic pets to counterfeit money. Beside spam post, some of the shops also appears in the darknet, which one can think it give them more "credibility" because of that.
These kind of scams are more common than you think and many people fall for it. Interpol and FBI posted recommendation how to protect yourself.

https://www.fbi.gov/scams-and-safety/common-scams-and-crimes/non-delivery-of-merchandise
This scams work even when illegal goods are being sold, they are less likely to be reported due to the person that purchased something he shouldn't, in the first place, might be in serious trouble.
From client perspective - buy such services/items in darknet from reputable vendors on legitimate marketplace. Try to verify every seller, use encryption and care of your OPSEC.